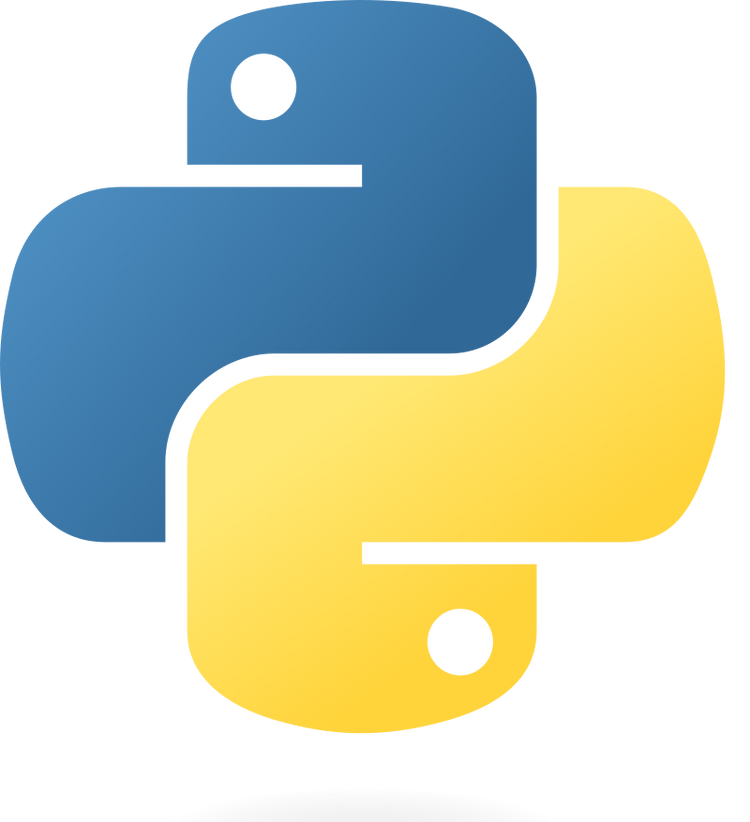
[Python] decorate
·
기타
Decorate는 특정 함수에 다른 함수를 추가하는 기능입니다. 이를 통해 함수를 간단하게 확장하고 재사용할 수 있습니다. 예시코드 def decorator_function(func): def wrapper(): print("데코레이터 함수가 호출되기 전입니다.") func() print("데코레이터 함수가 호출된 후입니다.") return wrapper @decorator_function def print_hello(): print("Hello") print_hello() # 출력 결과 # 데코레이터 함수가 호출되기 전입니다. # Hello # 데코레이터 함수가 호출된 후입니다.